๐พ โก๏ธ ๐ป Data to UI
Learning Objectives
When we build user interfaces we often take data and
๐ฝ๏ธ Cinema listings
Learning Objectives
Suppose you’re building a user interface to display the films that are now showing on a film website. We need to render some cinema listings in the user interface. Let’s define an acceptance criterion:
Given a list of film data
When the page first loads
Then it should display the list of films now showing, including the film title, times and film certificate
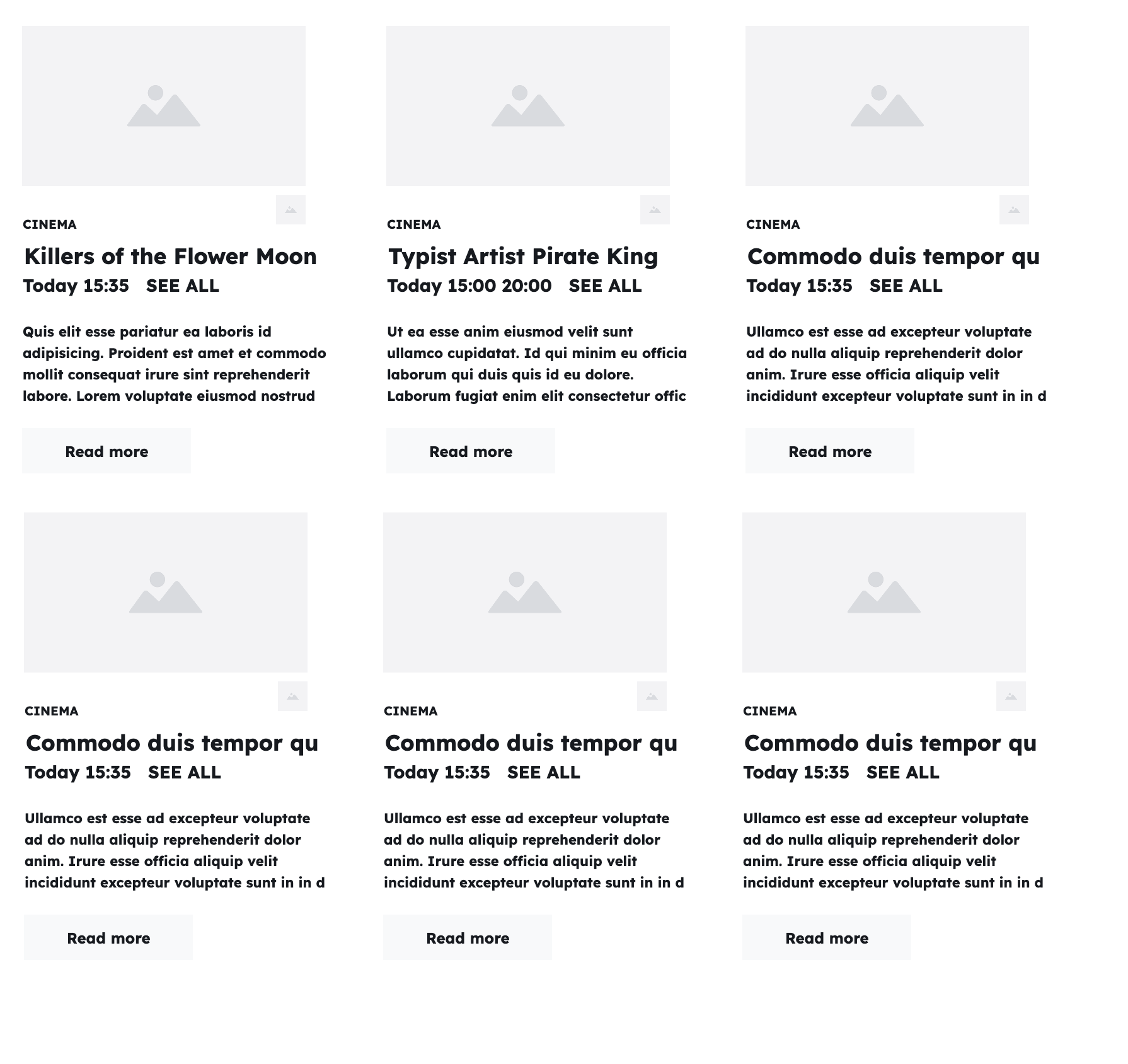
Here are some example film data:
const films = [
{
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
},
{
title: "Typist Artist Pirate King",
directory: "Carol Morley",
times: ["15:00", "20:00"],
certificate: "12A",
duration: 108,
},
];
To visualise the user interface, we can use a
Our task will be to build the film listings view from this list of data. Create an index.html file and follow along.
Youtube: Step-through-prep workshop ๐
๐ฝ Single datum
Learning Objectives
๐ฏ Sub-goal: Build a film card component
To break down this problem, we’ll render a single datum, before doing this for the whole list. Here’s one film:
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
Starting with this object, we’ll focus only on building this section of the user interface:
๐ผ๏ธ Open this wireframe of single film card
๐งฑ Composing elements
Learning Objectives
We can start by calling createElement
to create and
For now, we’ll only consider rendering the title
property from the film
object. Create this script in your index.html:
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
const filmTitle = document.createElement("h3");
filmTitle.textContent = film.title;
console.log(filmTitle);
If we open up the console tab, we should be able to see this element logged in the console. However, it won’t yet appear in the browser.
Uncaught ReferenceError: document is not defined
<!DOCTYPE html>
<html lang="en">
<head>
<title>Film View</title>
</head>
<body>
<script>
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
const filmTitle = document.createElement("h3");
filmTitle.textContent = film.title;
console.log(filmTitle);
</script>
</body>
</html>
Appending elements
To display the film card, we need to append it to another element that is already in the DOM tree. For now let’s append it to the body, because that always exists.
|
|
We can extend this card to include more information about the film by creating more elements:
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
const card = document.createElement("section");
const filmTitle = document.createElement("h3");
filmTitle.textContent = film.title;
card.append(filmTitle);
const director = document.createElement("p");
director.textContent = `Director: ${film.director}`;
card.append(director);
const duration = document.createElement("time");
duration.textContent = `${film.duration} minutes`;
card.append(duration);
const certificate = document.createElement("data");
duration.textContent = `Certificate: ${film.certificate}`;
card.append(certificate);
document.body.append(card);
Eventually, we will include all the information, to match the wireframe. This is a bit tedious, as we had to write lots of similar lines of code several times, but it works.
๐งผ Simplifying element creation
Learning Objectives
We now have a card showing all of the information for one film. The code we have is quite repetitive and verbose. It does similar things lots of times.
Let’s look at two ways we could simplify this code. First we will explore extracting a function. Then we’ll look at using <template>
tags.
Refactoring: Extracting a function
One way we can simplify this code is to refactor it.
Definition: refactoring
We can identify things we’re doing several times, and extract a function to do that thing for us.
In this example, we keep doing these three things:
- Create a new element (sometimes with a different tag name).
- Set that element’s text content (always to different values).
- Appending that element to some parent element (sometimes a different parent).
We could extract a function which does these three things. The things which are different each time need to be parameteres to the function.
We could write a function like this:
function createChildElement(parentElement, tagName, textContent) {
const element = document.createElement(tagName);
element.textContent = textContent;
parentElement.append(element);
return element;
}
And then rewrite our code to create the card like this:
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
function createChildElement(parentElement, tagName, textContent) {
const element = document.createElement(tagName);
element.textContent = textContent;
parentElement.append(element);
return element;
}
const card = document.createElement("section");
createChildElement(card, "h3", film.title);
createChildElement(card, "p", `Director: ${film.director}`);
createChildElement(card, "time", `${film.duration} minutes`);
createChildElement(card, "data", film.certificate);
document.body.append(card);
This code does exactly the same thing as the code we had before. By introducing a function we have introduced some advantages:
- Our code is smaller, which can make it easier to read and understand what it’s doing.
- If we want to change how we create elements we only need to write the new code one time, not for every element. We could add a class attribute for each element easily.
- We can see that each element is being created the same way. Before, we would have to compare several lines of code to see this. Because we can see they’re calling the same function, we know they’re made the same way.
There are also some drawbacks to our refactoring:
- If we want to change how we create some, but not all, elements, we may have made it harder to make these changes. When we want to include an image of the director, or replace the certificate text with a symbol, we will have to introduce branching logic.
- To follow how something is rendered, we need to look in a few places. This is something you will need to get used to, so it’s good to start practising now.
Deep dive: datetime
Stretch goal: Add the datetime
A <time>
element needs a datetime
attribute. Add this to the createChildElement
function to express the duration on the time element only.
PT1H52M
is the ISO 8601
format for 112
minutes.
๐ฑ Simplifying element creation
Learning Objectives
Using <template>
tags
We could simplify this code with a different technique for creating elements.
Until now, we have only seen one way to create elements: document.createElement
. The DOM has another way of creating elements - we can copy existing elements and then change them.
HTML has a useful tag designed to help make this easy, the <template>
tag. When you add a <template>
element to a page, it doesn’t get displayed when the page loads. It is an inert fragment of future HTML.
We can copy any DOM node, not just <template>
tags. For this problem, we will use a <template>
tag because it is designed for this purpose.
When we copy an element, its children get copied. This means we can write our template card as HTML:
<template id="film-card">
<section>
<h3>Film title</h3>
<p data-director>Director</p>
<time>Duration</time>
<p data-certificate>Certificate</p>
</section>
</template>
This is our template card. Place it in the body of your html. It doesn’t show up! Template HTML is like a wireframe; it’s just a plan. We can use this template to create a card for any film object. We will clone (copy) this template and populate it with data.
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
const card = document.getElementById("film-card").content.cloneNode(true);
// Now we are querying our cloned fragment, not the entire page.
card.querySelector("h3").textContent = film.title;
card.querySelector(
"[data-director]"
).textContent = `Director: ${film.director}`;
card.querySelector("time").textContent = `${film.duration} minutes`;
card.querySelector("[data-certificate]").textContent = film.certificate;
document.body.append(card);
This code will produce the same DOM elements in the page as the two other versions of the code we’ve seen (the verbose version, and the version using createChildElement
).
The first two approaches (the verbose version, and the createChildElement
version) did so by calling the same DOM functions as each other.
This approach uses different DOM functions. But it has the same effect.
Exercise: Consider the trade-offs
We’ve now seen two different ways of simplifying our function: extracting a function, or using a template tag.
Both have advantages and disadvantages.
Think of at least two trade-offs involved. What is better about the “extract a function” solution? What is better about the template tag solution? Could we combine them?
Share your ideas about trade-offs in a thread in Slack.
๐ Building a component
Learning Objectives
Recall our sub-goal:
๐ฏ Sub-goal: Build a film card component
Now that we have made a card work for one particular film, we can re-use that code to render any film object in the user interface with a general component. To do this, we wrap up our code inside a JavaScript function. JavaScript functions reuse code: so we can implement reusable UI components using functions.
const film = {
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
};
const createFilmCard = (template, film) => {
const card = template.content.cloneNode(true);
// Now we are querying our cloned fragment, not the entire page.
card.querySelector("h3").textContent = film.title;
card.querySelector("p").textContent = `Director: ${film.director}`;
card.querySelector("time").textContent = `${film.duration} minutes`;
card.querySelector("data").textContent = `Certificate: ${film.certificate}`;
// Return the card, rather than directly appending it to the page
return card;
};
const template = document.getElementById("film-card");
const filmCard = createFilmCard(template, film);
// Remember we need to append the card to the DOM for it to appear.
document.body.append(filmCard);
Exercise: Use destructuring
createFilmCard
function to use object destructuring in the parameters.๐ญ๐พ One to one
Learning Objectives
We can now render any one film data object in the UI. However, to fully solve this problem we must render a list of all of the film objects. For each film object, we need to render a corresponding film card in the UI. In this case, there is a
To create an array of card components, we can iterate through the film data using a for...of
loop:
const filmCards = [];
for (const item of films) {
filmCards.push(createFilmCard(item));
}
document.body.append(...filmCards);
// invoke append using the spread operator
However, there are alternative methods for building this array of UI components.
๐บ๏ธ Using map
Learning Objectives
We want to create a new array by applying a function to each element in the starting array. Earlier, we used a for...of
statement to apply the function createFilmCard
to each element in the array. However, we can also build an array using the map
array method. map
is a
map
. Then map
will use this function to create a new array.
Work through this map
exercise. It’s important to understand map before we apply it to our film data.
const arr = [5, 20, 30];
function double(num) {
return num * 2;
}
Our goal is to create a new array of doubled numbers given this array and function. We want to create the array [10, 40, 60]
. Look, it’s another “one to one mapping”
We are building a new array by applying double
to each item. Each time we call double
we store its return value in a new array:
function double(num) {
return num * 2;
}
const numbers = [5, 20, 30];
const doubledNums = [
double(numbers[0]),
double(numbers[1]),
double(numbers[2]),
];
But we want to generalise this. Whenever we are writing out the same thing repeatedly in code, we probably want to make a general rule instead. We can do this by calling map
:
|
|
Use the array visualiser to observe what happens when map
is used on the arr
. Try changing the elements of arr
and the function that is passed to map
. Answer the following questions in the visualiser:
- What does
map
do? - What does
map
return? - What parameters does the
map
method take? - What parameters does the callback function take?
Play computer with the example to see what happens when the map
is called.
Given the list of film data:
const films = [
{
title: "Killing of Flower Moon",
director: "Martin Scorsese",
times: ["15:35"],
certificate: "15",
duration: 112,
},
{
title: "Typist Artist Pirate King",
director: "Carol Morley",
times: ["15:00", "20:00"],
certificate: "12A",
duration: 108,
},
];
Use createFilmCard
and map
to create an array of film card components. In a local project, render this array of components in the browser.
Prep Teamwork Project
๐ค๐ฝ FeedbackLearning Objectives
Preparation
Your team must be defined beforehand and be composed of the following:
- A mix of technical skills/levels
- A mix of genders
- Maximum 5 members
To do so organise yourselves as a cohort.
Tip: try to work with people you haven’t worked with yet.
Introduction
Working as a team when developing software is very important. The collaboration, knowledge sharing and diversity of skill sets result in higher-quality products, faster development cycles, and a more efficient workflow. This delivers success in meeting customer needs and achieving project goals.
During the JS3 module, you will prepare a Teamwork Project. You will be assigned to a team by the volunteers. You will work as a team on a fictional digital product for a fictional client. You are not going to do any coding. This project aims to improve your teamwork skills. You will learn how to get prepared for a product before the software development phase.
You will:
- Week 1: Decide how you are going to work as a team
- Week 2: Define the minimum viable product for your project
- Week 3: Define features and user stories for your product
- Week 4: Present a brief for your product and your teamwork
Each team member should:
- Organise and attend calls
- Have clear responsibilities
- Help each other when needed
Understand team roles
๐ฏ Goal: Reflect about team roles (30 minutes)
This is an individual task.
- Read the article “Belbin’s Team Roles” and watch the video.
- Reflect on the team roles you have taken so far in your life.
Keep this article in mind if you need to change your behaviour to help your team perform better during the project.
Prepare for your team meeting
๐ฏ Goal: Be prepared for the first meeting with your team (30 minutes)
One of you must organise the meeting. So agree or volunteer for it. This should take you around 15 min.
Prepare for the first call with your team. Consider the below aspects (15 min)
- Your understanding of the project
- Your questions
- What you think you as a team should prepare for
PS: Do NOT discuss the day plan exercises. Otherwise, this will disrupt the lesson.
Have a first meeting with your team
๐ฏ Goal: Understand the teamwork project (60 minutes)
Agenda for this first meeting:
Discuss your understanding of the project
Discuss everyone’s questions
Define what you think you as a team should prepare for
PS: Do NOT discuss the day plan exercises. Otherwise, this will disrupt the lesson.